Properties of the drag
& drop elements |
Data type, [r] read-only,
[rw] read and write |
h 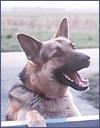 |
number [r] | |
Current height. A complete example line how to
use a property: alert(dd.elements.rex.h);
|
|
Retrieves the name (images) or ID (layers) which has
been passed to SET_DHTML(). alert(dd.elements.rex.name);
|
|
Current width of image/layer. Use
resizeTo() or resizeBy() to alter the
size. alert(dd.elements['rex'].w);
|
|
Current X position inside the document. x and y are
related to the left top corner of the document/page. Use
moveTo() or moveBy() to change the
element's position. alert(dd.elements.rex.x);
|
|
Current Y position. alert(dd.elements["rex"].y);
|
|
Current background color of a layer. Use the method
setBgColor() to change the color. |
children |
object array
[r] | |
Contains references to the dhtml & drag&drop
enabled children of the element, usually images
(= children) nested via HTML into a layer
(= parent element). Note that the
addChild() method allows arbitrary items to be
added to the children array, i.e. to be handled
as if they were nested into the element. Children
automatically follow their parent's drag operations,
moveTo(), moveBy(), hide(),
show() and
maximizeZ() methods, provided that neither the
DETACH_CHILDREN command
nor the .detachChild() method has been applied
to the parent. Examples for feasible
statements:
dd.elements.layerName.children[i] or
dd.elements.layerName.children["imageName"]
or
dd.elements["layerName"].children.imageName
and so forth.
dd.elements.layerName.children.length
retrieves the number of images contained within the
layer. |
|
This array contains the copies of a drag drop image
which has been multiplied by the COPY command
or the copy() method. With
dd.elements.imgName.copies[i] you'll get
a reference to copy number i of "imgName" (first copy
has the index 0, as usual with arrays).
dd.elements.imgName.copies.length
retrieves the number of copies. Being a
fully-equipped drag drop image by its own, each of the
copies internally gets its own name, which is the name
of the original image with a number appended, beginning
with 1 for the first copy. Therefore
dd.elements.imgName.copies[3] is also
accessible via dd.elements.imgName4.
dd.elements.muckl.copies[].resizeTo(,); Hint ;-) :
Original size was (100, 130) |
|
Initial (default) height. |
|
For images only. This property contains the default
URL (initial path) even after the image has been
swapped. For example, you might write
dd.elements.myImg.swapImage(dd.elements.myImg.defsrc);
to restore the original source of "myImg". |
|
Initial (default) width. |
|
Default X position. The library uses these default
co-ordinates to re-integrate images or relatively
positioned layers into the logical structure of the
page/text flow after the window or a layer has been
resized. You might read these default co-ordinates to
find out where a dragged item actually belongs to.
Example how to move an item to its default position:
var my_item = dd.elements["itemName"];
my_item.moveTo(my_item.defx, my_item.defy);
|
|
Original (default) Y position. |
|
Original (default) z-index. Used by the library to
restore the z-index of an element to which the RESET_Z
command
has been applied. You might modify the default stacking
order of your Drag&Drop elements by changing their
defz values. |
|
Direct reference to the DOM node of the item.
Increases the flexibility of the API essentially.
Example:
dd.elements.someName.div.ondblclick = myDblClickFunc;
This example shows how to assign a doubleclick
eventhandler to a DHTML & Drag&Drop
element. Please recall, however, that you should never
directly change properties which are managed and
accessible through the API of wz_dragdrop.js, such as
position, z-index or size! For example, do not write
dd.elements.someName.div.width = 314px;. Use
resizeTo() instead. |
|
Indicates whether the item can be dragged
horizontally only. To toggle this movement restriction,
you can either apply the HORIZONTAL command,
or invoke the setHorizontal() method. (See
also vertical.) |
|
Index of item within the dd.elements array.
Useful, for example, to map the draggable items directly
on an array of formular inputs:
document.myForm.elements[dd.obj.index].value
= dd.obj.x; dd.obj is a
reference to the currently active item, see further down
this page: |
|
true, if element is an image,
false if it's a layer. Example:
if
(dd.elements[3].is_image) {
dd.elements[3].swapImage('images/anotherImg.jpg'); }
|
maxoffl, maxofft, maxoffr, maxoffb |
number [rw] | |
These properties reflect the values of the
MAXOFFLEFT/MAXOFFTOP/MAXOFFRIGHT/MAXOFFBOTTOM-commands,
that is they limit how far the element can be dragged
away from its default position. Being writable (rw),
these properties can directly be set or changed at
runtime. A value of -1 means unlimited drag range.
|
maxw,
maxh, minw, minh |
number [rw] | |
Reflecting the values of the
MAXWIDTH/MAXHEIGHT/MINWIDTH/MINHEIGHT-commands,
these properties limit how far the element can be
resized with the mouse. Only relevant for elements the
RESIZABLE command has been applied to. Being writable
(rw), these properties can directly be set or changed at
runtime. A value of -1 means unlimited resizability.
|
|
Exists only with images which are copies. This
property is a reference to the original, i.e. the
drag&drop image from which the copy has been
derived. Example: if
(dd.obj.original) {
dd.obj.original.swapImage('images/anotherImg.jpg'); }
By looking for the property original, these
lines examine if the currently dragged element
(dd.obj) is a copy. If yes, its original image
(original) is swapped. |
|
This property exists only for elements which are
children of another element, for example for images
nested into a layer. Inversely to the children
array, parent is a reference from a child to its parent.
With if(dd.elements.imageName.parent)...;
or if(dd.obj.parent)...; , for
example, you might examine if a certain image (first
example) or the currently dragged item (second example)
belongs to a layer (or to another image) or not. |
|
Images only. Current URL (path, source). To swap an
image, use its swapImage() method. |
|
Layers only. Current inner HTML that has been
written to the layer by use of the write()
method. |
|
Indicates whether the item can be dragged vertically
only. To toggle this movement restriction, you can
either apply the VERTICAL command,
or invoke the setVertical() method. (See
also horizontal.) |
|
Indicates visibility of item. true if
element is visible, false if element has been
hidden by use of the .hide() method. To toggle
the visibility of an item, it's strictly recommended to
use its hide() and show() methods
only. |
|
Retrieves the current z-index, i. e. the stacking
order relative to the other drag-drop elements.
Z-indices are internally managed by wz_dragdrop.js and
usually not of interest for external scripting use.
Otherwise, use the method setZ() to set the z-index of
the item. Or maximizeZ() to lift the element to the
currently highest z-index. |
Methods of the Drag
& Drop Elements |
copy()
|
For images only. Creates a copy and adds it to the
image's copies array. Optionally,
copy() accepts a parameter that specifies how
many copies to be created: dd.elements.cat.copy(2);
|
hide() |
Hides item: dd.elements.race.hide();.
Optionally, hide() accepts a parameter (value:
true) which completely removes the empty space (spacer)
for the hidden item: dd.elements.race.hide(true);
It's strictly recommended to toggle the visibility
of items with hide() and show() only.
For example, to hide certain items on your page
initially, invoke their hide() methods
immediately after SET_DHTML() has been called, even if
the 'visibility' CSS attributes of these items have been
set to 'hidden' in advance. dd.elements.race.show();
alert(dd.elements.race.visible);
|
moveBy(deltaX,deltaY) |
Often more convenient than moveTo(). dd.elements.einstein.moveBy(+20, -7);
|
moveTo(new_x, new_y)
|
Moves element to the document-related co-ordinates
newX, newY. var einstein =
dd.elements.einstein; einstein.moveTo(einstein.x-20,
einstein.y+7); |
resizeBy(deltaW,deltaH)
|
Often more convenient than resizeTo(). dd.elements.einstein.resizeBy(22, -5);
|
resizeTo(width,height)
|
Adjusts width and height of a drag&drop layer or
image to the specified pixel sizes. Warning, regarding
only layers, not images: Some browsers with less
extended DHTML abilities (Netscape 4, Opera <7)
are not able to re-flow the text within a layer after
its size has been changed. Therefore, if the remaining
space inside the layer is insufficient, it may happen
that parts of the text become clipped off and invisible.
dd.elements.einstein.resizeTo(,); |
show() |
Makes item visible again. dd.elements.race.show();
|
swapImage("new_path") |
For images only. Replaces an image by the specified
path that must be enclosed in single- or double-quotes.
dd.elements.fateba_se.swapImage('ko5.jpg');
dd.elements.fateba_se.swapImage(dd.elements.fateba_se.defsrc);
To swap a multiplied (through the COPY-command
or the copy() method) image and any of his
copies ad once, swapImage() accepts an optional second
parameter true. |
addChild(image or
layer)
|
Adds the item specified by the parameter to the
element's children array. Hence the new 'child'
will be treated as if being nested into the parent
element, and be dependent from the parent's behavior,
i.e. movements and visibility status.
addChild() can even 'steal' items
from other elements, and automatically remove them from
the previous parent's children array. However,
addChild() can not steal DIVs which were
natively nested into another parent layer, i.e. through
the HTML code. Tip: addChild()
can even build parent-child relationships between
images, see example on the left side. dd.elements.fateba_se.addChild(dd.elements.ko5_st);
As parameter, addChild() accepts
either the name of the new child in single or double
quotes, or a reference to the item as in the example
above. |
attachChild(child
element) |
For layers that contain child elements (images or
DIVs) that have previously been made independent from
the layer through the DETACH_CHILDREN command or the
detachChild() method. As parameter,
attachChild() requires one of these children
(i.e. an element of the layer's children
array). attachChild() renders this child dependent from
the layer's behavior again (i.e. from the layer's
moveTo(), moveBy(), hide() and show() methods). Other
than addChild(), attachChild() is not
capable of 'stealing' an image or DIV from elsewhere and
adding it to the layer's own children array.
Parameter: either the name of the image in single or
double quotes, or the index of the image in the layer's
children array, or a reference to the image as
in the following example: dd.elements.fateba_se.attachChild(dd.elements.ko5_st);
|
detachChild(child
element) |
dd.elements.fateba_se.detachChild('ko5_st');
For layers containing child elements - child
elements are either images nested into a DIV, or
elements (images or DIVs) turned into children of the
layer via addChild(). Requires one of these
children as parameter, and detaches it from the layer,
i.e. makes it's movements and visibility independent
from the layer. This method has the same effect for a
certain element, as the DETACH_CHILDREN command, passed
to SET_DHTML(), would have for all images being nested
into the layer. Parameter: equivalent to
.attachChild() |
del() |
Removes the element, that is, disables its DHTML
capabilities and its draggability and removes it from
the dd.elements array. However, del() does not
destroy the HTML element per se. Example:
dd.elements.someName.del(); |
getEltBelow() |
The API's hit-test function. Returns the element
below the concerned item, that is, the topmost element
overlapped by the concerned item, otherwise
null. Might be used, for instance, in
my_DropFunc() to get directly the item on which
the dragged element (referencable by dd.obj)
has been dropped:
var dropTarget =
dd.obj.getEltBelow(); if(dropTarget != null)
alert(dropTarget.name);
To satisfy the
"hit" condition, the element below must be in both
dimensions overlapped by at least 50%. |
maximizeZ() |
Sets the z-index of the element to the currently
highest level. Therefore this element will be able to
overlap each other. Note: This method automatically is
performed if the element is hit by a mousedown-event,
i. e. selected to become current drag object. |
setBgColor("color") |
Changes the background color of a layer, or a
transparent Drag'nDrop image (GIF, PNG) (!), to the
value specified by the parameter. dd.elements.bluelyr.setBgColor(""); |
setCursor("CSS
value") |
Alters cursor over concerned Drag&Drop item.
Accepts as parameter either a CSS cursor value as
string, for example
dd.elements.someImg.setCursor('crosshair');, or
alternatively one of the API's cursor commands,
for example
dd.elements.someImg.setCursor(CURSOR_CROSSHAIR); |
setDraggable(true/false)
|
Parameter true enables, false
disables draggability of the element. However, even with
switched-off draggability, the element is not removed
from the dd.elements array, hence keeping any
of its DHTML abilities, except of the ability to be
dragged or resized with the mouse. dd.elements.dog.setDraggable(false);
dd.elements.dog.setDraggable(true);
|
setHorizontal(true/false)
|
After calling that method with the parameter
true, the item can be dragged horizontally
only. The parameter false removes that
restriction again. Compare with the HORIZONTAL command
which has the same effect, but can only be applied with
the intial call of SET_DHTML(). The current
state can be checked via the property
horizontal. Play again with the dog Teddy (or:
learn how to flip a slider): dd.elements.dog.setHorizontal(true);
dd.elements.dog.setHorizontal(false);
dd.elements.dog.setVertical(true);
dd.elements.dog.setVertical(false);
|
setOpacity(float)
|
Sets the opacity of the item (image or DIV).
Parameter must be a decimalpoint number between 0.0
(entirely transparent, invisible) and 1.0 (opaque, no
transparency). This method takes effect in Gecko
browsers (Mozilla, Firefox etc.) and in Windows-IE.
dd.elements.dog.setOpacity(
); |
setResizable(true/false)
|
Enables/disables RESIZABLE functionality of
concerned element. RESIZABLE functionality means that
the element can be resized instead of dragged if the
SHIFT key is pressed during a drag movement (more
precisely: at the beginning of a drag action).
dd.elements.dog.setResizable(false);
dd.elements.dog.setResizable(true);
|
setScalable(true/false)
|
Enables/disables SCALABLE functionality of element.
Resembling RESIZABLE in any other aspect, SCALABLE
preserves the width/height ratio of item. dd.elements.dog.setScalable(false);
dd.elements.dog.setScalable(true);
|
setVertical(true/false)
|
Has the same effect as the VERTICAL command,
but allows you to toggle that dragdirection restriction
at runtime. The current state can be checked via the
property vertical. See also
setHorizontal(). |
setZ(number) |
Sets the z-index of the element to the specified
value. May be useful, for example, to permute the
stacking order of two drag drop items overlapping each
other - the property z retrieves the current z-index.
|
write("html")
|
Writes to a layer and replaces its content. Plus,
assigns the new HTML to the text property of
the concerned Drag&Drop&DHTML object. Drag 'n
Drop images within the layer, however, are protected and
will not be deleted. dd.elements.bluelyr.write("
"); |
Further interfaces to
the library |
Properties and methods of
the library's main object dd ("drag-drop"): |
dd.elements |
Array of drag drop elements. To access a certain
drag drop element you may use any of the following
notations: dd.elements.ItemName
or dd.elements["ItemName"]
or dd.elements[i] where i is
index of the element.
dd.elements.length returns
the number of drag drop items on your page (including
copies of multiplied images). |
dd.obj |
Contains the current drag object; otherwise nothing
or null. For example, to find out if "image2" is current
drag object, simply write:
if (dd.obj == dd.elements.image2) {dependent statements;}
or
alternatively if (dd.obj.name == "image2") {dependent statements;}
An easy and direct way to access a
property or call a method of the current drag item is a
statement like dd.obj.property or
dd.obj.method() |
dd.getScrollX() |
Returns how far the window has been scrolled
horizontally. alert(dd.getScrollX());
|
dd.getScrollY() |
Returns how far the window has been scrolled
vertically. alert(dd.getScrollY());
|
dd.getWndH() |
Returns the inner window height (of the visible
client area). alert(dd.getWndH());
|
dd.getWndW() |
Returns the inner window width (of the visible
client area). alert(dd.getWndW());
|
dd.Int() |
Transforms argument surely into integer. Even if the
transformation actually isn't possible, as for
dd.Int("abc"), the number 0 will be returned. This
behavior avoids data type errors. |
dd.db |
= document.body, or, in Non-BackCompat-mode of IE6,
document.documentElement. |
dd.ie |
true, if Internet Explorer 4+ |
dd.n |
navigator.userAgent (lower case). alert(dd.n);
|
dd.n4 |
true, if Netscape 4 (not Netscape > 4). |
dd.n6 |
true, if browser based on Gecko-Engine (Mozilla,
Netscape 6+, Galeon, Phoenix...); also true with
browsers with advanced W3C-DOM support. |
dd.op |
true, if Opera 5+ browser, else false. |
Making layers draggable
by way of addition |
ADD_DHTML()
|
Converts layers (DIV elements) into DHTML Drag'nDrop
elements by way of addition, even after the page has
fully loaded. Useful particularly for dynamically
created layers. Note that SET_DHMTL() must have been
invoked while the page was still loading, optionally
even without arguments. ADD_DHTML() does not work with
images. For a workaround you might insert images each
into a DIV. Like with SET_DHTML(),
ADD_DHTML() requires the ID of the concerned layer(s) as
parameter(s), each enclosed in single or double quotes,
and you can append individual commands with a plus-sign.
Of course, each of these layers will be added to the
dd.elements array and hence provide the same
properties and methods as layers initialized via
SET_DHTML(). Example: ADD_DHTML("NewLayer1"+VERTICAL+MAXOFFTOP+100+MAXOFFBOTTOM+100);
ADD_DHTML("NewLayer2"+HORIZONTAL);
dd.elements.NewLayer1.setBgColor("#99dd99");
dd.elements.NewLayer2.moveBy(16,8);
|